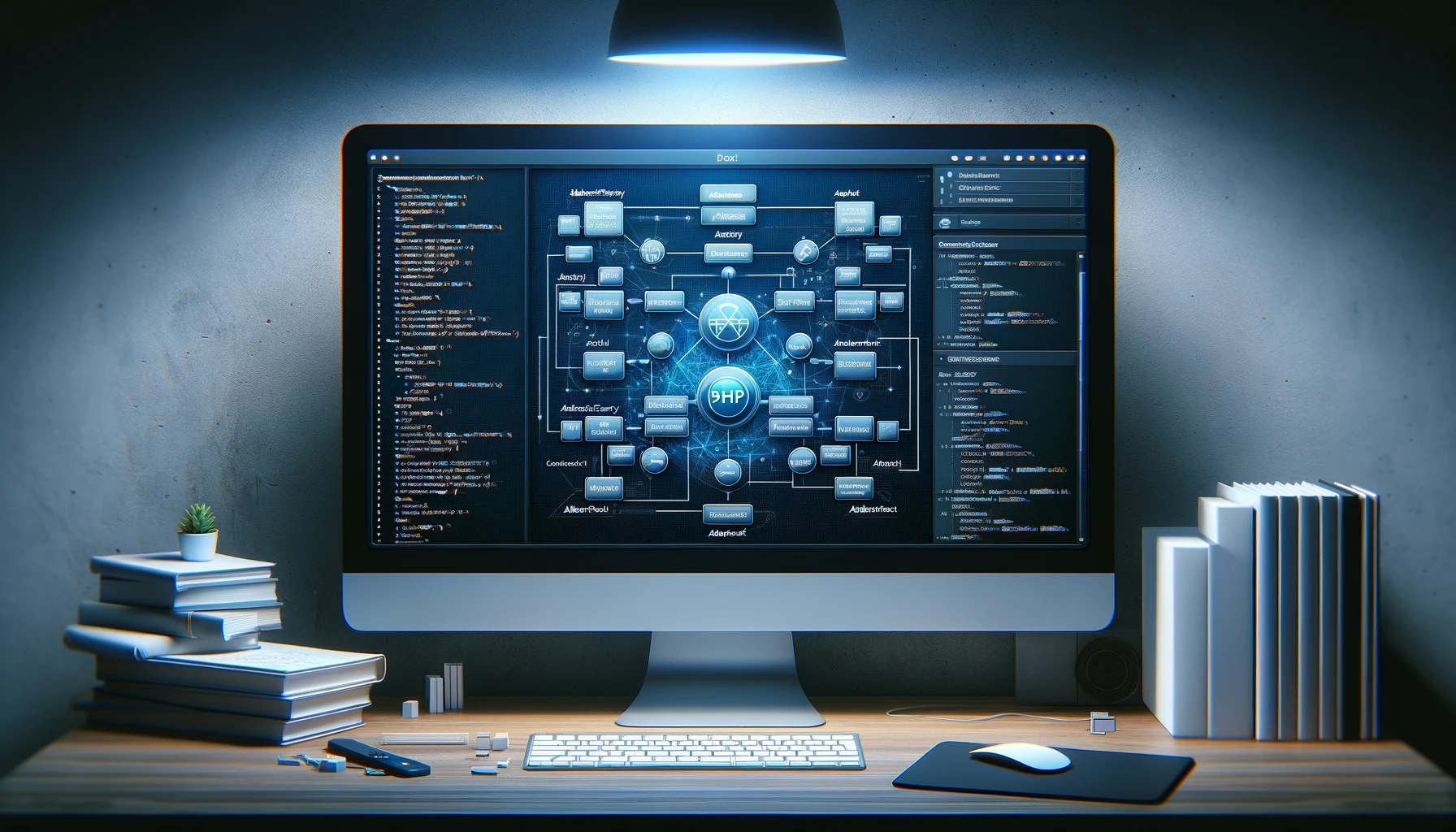
Abstract Factory Pattern in PHP
Sam Ciaramilaro • December 13, 2022
application-designThe Abstract Factory Pattern is a design pattern that provides a way to encapsulate a group of individual factories that have a common theme. The Abstract Factory Pattern allows a program to create multiple families of related objects without specifying their concrete classes. The pattern also allows a program to work with objects of different families without having to know their concrete classes.
The Abstract Factory Pattern is part of the creational design patterns family, and is often used to create a set of related objects, or families of objects. It is also useful for isolating system dependencies and for building flexible systems that can be easily extended. The Abstract Factory Pattern encourages low coupling between objects, and helps to prevent a system from becoming overly complex.
The Abstract Factory Pattern is made up of four main components:
The Abstract Factory: This provides an interface for creating the related objects.
The Concrete Factory: This is the concrete implementation of the Abstract Factory which provides specific implementations of the Abstract Factory's methods.
The Abstract Product: This is an interface that defines the product that the Abstract Factory will create.
The Concrete Product: This is the concrete implementation of the Abstract Product which provides specific implementations of the Abstract Product's methods.
The Abstract Factory Pattern is a useful pattern for creating families of objects that are related and have common characteristics. It allows a program to create objects from different families without knowing the exact type of object that is being created. This helps to keep the code flexible and makes it easier to change the implementation of the objects without affecting the code.
The Abstract Factory Pattern can be implemented in many different programming languages, but in this article we will focus on the implementation using PHP.
The following example shows how to create a basic Abstract Factory Pattern using PHP.
// Define the Abstract Factory class
abstract class AbstractFactory {
abstract public function createProductA();
abstract public function createProductB();
}
// Define the Concrete Factory class
class ConcreteFactory extends AbstractFactory {
public function createProductA() {
return new ConcreteProductA();
}
public function createProductB() {
return new ConcreteProductB();
}
}
// Define the Abstract Product class
abstract class AbstractProduct {
abstract public function getName();
}
// Define the Concrete Product classes
class ConcreteProductA extends AbstractProduct {
public function getName() {
return 'Product A';
}
}
class ConcreteProductB extends AbstractProduct {
public function getName() {
return 'Product B';
}
}
// Create the Concrete Factory
$factory = new ConcreteFactory();
// Create the products
$productA = $factory->createProductA();
$productB = $factory->createProductB();
// Output the products
echo $productA->getName();
echo $productB->getName();
In this example, we have created an Abstract Factory class which defines an interface for creating the related objects. We have then created a Concrete Factory class which extends the Abstract Factory class and provides specific implementations for creating the related objects.
We have also defined an Abstract Product class which defines an interface for the products that the factory will create, and two Concrete Product classes which provide specific implementations of the Abstract Product class. Finally, we have created an instance of the Concrete Factory and used it to create two products, Product A and Product B. We have then outputted the names of the two products to demonstrate that the Abstract Factory Pattern is working as expected.
The Abstract Factory Pattern is a powerful design pattern that allows a program to create multiple families of related objects without specifying their concrete classes. It is a useful pattern for creating flexible systems that can easily be extended, and for isolating system dependencies. It also encourages low coupling between objects, and helps to prevent a system from becoming overly complex.